mirror of
https://github.com/planetmint/planetmint-go.git
synced 2025-07-05 20:22:33 +00:00
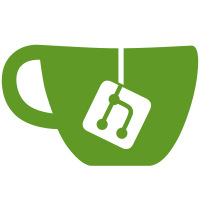
* added a bit of logging * added update messages to mqtt (every second with a timestamp) * added isConnectionOpen to the interface and analysis this during execution * added connection loss handler and status check * put some global objects into an objects to avoid inter service testing issues * TestingLocker Mutex to RWMutex (increase performance) * termintationMutex became a sync.RWMutex * added logging to the mqtt mock client * made monitor Mutex a RWMutex * added Mutex protection to the numberOfElements varialbe * added another Waiting block to the machine attestation methods (CI tests) * had to adjust the test cases to the impact of that change. Signed-off-by: Jürgen Eckel <juergen@riddleandcode.com>
117 lines
2.8 KiB
Go
117 lines
2.8 KiB
Go
package e2e
|
|
|
|
import (
|
|
"errors"
|
|
"strings"
|
|
|
|
"github.com/cosmos/cosmos-sdk/crypto/hd"
|
|
"github.com/cosmos/cosmos-sdk/crypto/keyring"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
banktypes "github.com/cosmos/cosmos-sdk/x/bank/types"
|
|
"github.com/planetmint/planetmint-go/lib"
|
|
clitestutil "github.com/planetmint/planetmint-go/testutil/cli"
|
|
"github.com/planetmint/planetmint-go/testutil/moduleobject"
|
|
"github.com/planetmint/planetmint-go/testutil/network"
|
|
"github.com/planetmint/planetmint-go/testutil/sample"
|
|
machinetypes "github.com/planetmint/planetmint-go/x/machine/types"
|
|
)
|
|
|
|
func CreateAccount(network *network.Network, name string, mnemonic string) (account *keyring.Record, err error) {
|
|
val := network.Validators[0]
|
|
|
|
kb := val.ClientCtx.Keyring
|
|
account, err = kb.NewAccount(name, mnemonic, keyring.DefaultBIP39Passphrase, sample.DefaultDerivationPath, hd.Secp256k1)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return account, nil
|
|
}
|
|
|
|
func FundAccount(network *network.Network, account *keyring.Record, tokenDenom string) (err error) {
|
|
val := network.Validators[0]
|
|
|
|
addr, err := account.GetAddress()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
// sending funds to account to initialize account on chain
|
|
coin := sdk.NewCoins(sdk.NewInt64Coin(tokenDenom, 10000))
|
|
msg := banktypes.NewMsgSend(val.Address, addr, coin)
|
|
out, err := lib.BroadcastTxWithFileLock(val.Address, msg)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
err = network.WaitForNextBlock()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
err = network.WaitForNextBlock()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
rawLog, err := clitestutil.GetRawLogFromTxOut(val, out)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
if !strings.Contains(rawLog, "cosmos.bank.v1beta1.MsgSend") {
|
|
err = errors.New("failed to fund account")
|
|
}
|
|
|
|
return
|
|
}
|
|
|
|
func AttestMachine(network *network.Network, name string, mnemonic string, num int, tokenDenom string) (err error) {
|
|
val := network.Validators[0]
|
|
|
|
account, err := CreateAccount(network, name, mnemonic)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
err = FundAccount(network, account, tokenDenom)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
// register Ta
|
|
prvKey, pubKey := sample.KeyPair(num)
|
|
ta := moduleobject.TrustAnchor(pubKey)
|
|
registerMsg := machinetypes.NewMsgRegisterTrustAnchor(val.Address.String(), &ta)
|
|
_, err = lib.BroadcastTxWithFileLock(val.Address, registerMsg)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
err = network.WaitForNextBlock()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
addr, err := account.GetAddress()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
machine := moduleobject.Machine(name, pubKey, prvKey, addr.String())
|
|
attestMsg := machinetypes.NewMsgAttestMachine(addr.String(), &machine)
|
|
_, err = lib.BroadcastTxWithFileLock(addr, attestMsg)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
err = network.WaitForNextBlock()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
err = network.WaitForNextBlock()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return
|
|
}
|