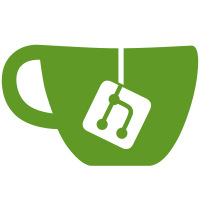
This bugfix implements correct way to calculate source sha1 hash, before it will changed, by propagation of unchanged sources. This commit will also include regenerated static files and *.hash files.
161 lines
5.1 KiB
Plaintext
Generated
161 lines
5.1 KiB
Plaintext
Generated
<!DOCTYPE html>
|
|
<html>
|
|
<head>
|
|
<meta charset="utf-8">
|
|
<title>Go by Example: Channel Buffering</title>
|
|
<link rel=stylesheet href="site.css">
|
|
</head>
|
|
<script>
|
|
onkeydown = (e) => {
|
|
|
|
if (e.key == "ArrowLeft") {
|
|
window.location.href = 'channels';
|
|
}
|
|
|
|
|
|
if (e.key == "ArrowRight") {
|
|
window.location.href = 'channel-synchronization';
|
|
}
|
|
|
|
}
|
|
</script>
|
|
<body>
|
|
<div class="example" id="channel-buffering">
|
|
<h2><a href="./">Go by Example</a>: Channel Buffering</h2>
|
|
|
|
<table>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>By default channels are <em>unbuffered</em>, meaning that they
|
|
will only accept sends (<code>chan <-</code>) if there is a
|
|
corresponding receive (<code><- chan</code>) ready to receive the
|
|
sent value. <em>Buffered channels</em> accept a limited
|
|
number of values without a corresponding receiver for
|
|
those values.</p>
|
|
|
|
</td>
|
|
<td class="code empty leading">
|
|
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
<a href="http://play.golang.org/p/3BRCdRnRszb"><img title="Run code" src="play.png" class="run" /></a><img title="Copy code" src="clipboard.png" class="copy" />
|
|
<div class="highlight"><pre><span class="kn">package</span> <span class="nx">main</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre><span class="kn">import</span> <span class="s">"fmt"</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre><span class="kd">func</span> <span class="nx">main</span><span class="p">()</span> <span class="p">{</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>Here we <code>make</code> a channel of strings buffering up to
|
|
2 values.</p>
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre> <span class="nx">messages</span> <span class="o">:=</span> <span class="nb">make</span><span class="p">(</span><span class="kd">chan</span> <span class="kt">string</span><span class="p">,</span> <span class="mi">2</span><span class="p">)</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>Because this channel is buffered, we can send these
|
|
values into the channel without a corresponding
|
|
concurrent receive.</p>
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre> <span class="nx">messages</span> <span class="o"><-</span> <span class="s">"buffered"</span>
|
|
<span class="nx">messages</span> <span class="o"><-</span> <span class="s">"channel"</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>Later we can receive these two values as usual.</p>
|
|
|
|
</td>
|
|
<td class="code">
|
|
|
|
<div class="highlight"><pre> <span class="nx">fmt</span><span class="p">.</span><span class="nx">Println</span><span class="p">(</span><span class="o"><-</span><span class="nx">messages</span><span class="p">)</span>
|
|
<span class="nx">fmt</span><span class="p">.</span><span class="nx">Println</span><span class="p">(</span><span class="o"><-</span><span class="nx">messages</span><span class="p">)</span>
|
|
<span class="p">}</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
</table>
|
|
|
|
<table>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code">
|
|
|
|
<div class="highlight"><pre><span class="gp">$</span> go run channel-buffering.go
|
|
<span class="go">buffered</span>
|
|
<span class="go">channel</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
</table>
|
|
|
|
|
|
<p class="next">
|
|
Next example: <a href="channel-synchronization">Channel Synchronization</a>.
|
|
</p>
|
|
|
|
<p class="footer">
|
|
by <a href="https://markmcgranaghan.com">Mark McGranaghan</a> | <a href="https://github.com/mmcgrana/gobyexample/blob/master/examples/channel-buffering">source</a> | <a href="https://github.com/mmcgrana/gobyexample#license">license</a>
|
|
</p>
|
|
</div>
|
|
<script>
|
|
var codeLines = [];
|
|
codeLines.push('');codeLines.push('package main\u000A');codeLines.push('import \"fmt\"\u000A');codeLines.push('func main() {\u000A');codeLines.push(' messages := make(chan string, 2)\u000A');codeLines.push(' messages \x3C- \"buffered\"\u000A messages \x3C- \"channel\"\u000A');codeLines.push(' fmt.Println(\x3C-messages)\u000A fmt.Println(\x3C-messages)\u000A}\u000A');codeLines.push('');
|
|
</script>
|
|
<script src="site.js" async></script>
|
|
</body>
|
|
</html>
|