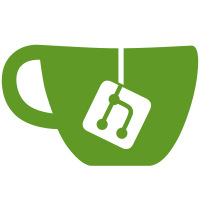
This bugfix implements correct way to calculate source sha1 hash, before it will changed, by propagation of unchanged sources. This commit will also include regenerated static files and *.hash files.
176 lines
5.5 KiB
Plaintext
Generated
176 lines
5.5 KiB
Plaintext
Generated
<!DOCTYPE html>
|
|
<html>
|
|
<head>
|
|
<meta charset="utf-8">
|
|
<title>Go by Example: Channels</title>
|
|
<link rel=stylesheet href="site.css">
|
|
</head>
|
|
<script>
|
|
onkeydown = (e) => {
|
|
|
|
if (e.key == "ArrowLeft") {
|
|
window.location.href = 'goroutines';
|
|
}
|
|
|
|
|
|
if (e.key == "ArrowRight") {
|
|
window.location.href = 'channel-buffering';
|
|
}
|
|
|
|
}
|
|
</script>
|
|
<body>
|
|
<div class="example" id="channels">
|
|
<h2><a href="./">Go by Example</a>: Channels</h2>
|
|
|
|
<table>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p><em>Channels</em> are the pipes that connect concurrent
|
|
goroutines. You can send values into channels from one
|
|
goroutine and receive those values into another
|
|
goroutine.</p>
|
|
|
|
</td>
|
|
<td class="code empty leading">
|
|
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
<a href="http://play.golang.org/p/MaLY7AiAkHM"><img title="Run code" src="play.png" class="run" /></a><img title="Copy code" src="clipboard.png" class="copy" />
|
|
<div class="highlight"><pre><span class="kn">package</span> <span class="nx">main</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre><span class="kn">import</span> <span class="s">"fmt"</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre><span class="kd">func</span> <span class="nx">main</span><span class="p">()</span> <span class="p">{</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>Create a new channel with <code>make(chan val-type)</code>.
|
|
Channels are typed by the values they convey.</p>
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre> <span class="nx">messages</span> <span class="o">:=</span> <span class="nb">make</span><span class="p">(</span><span class="kd">chan</span> <span class="kt">string</span><span class="p">)</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p><em>Send</em> a value into a channel using the <code>channel <-</code>
|
|
syntax. Here we send <code>"ping"</code> to the <code>messages</code>
|
|
channel we made above, from a new goroutine.</p>
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre> <span class="k">go</span> <span class="kd">func</span><span class="p">()</span> <span class="p">{</span> <span class="nx">messages</span> <span class="o"><-</span> <span class="s">"ping"</span> <span class="p">}()</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>The <code><-channel</code> syntax <em>receives</em> a value from the
|
|
channel. Here we’ll receive the <code>"ping"</code> message
|
|
we sent above and print it out.</p>
|
|
|
|
</td>
|
|
<td class="code">
|
|
|
|
<div class="highlight"><pre> <span class="nx">msg</span> <span class="o">:=</span> <span class="o"><-</span><span class="nx">messages</span>
|
|
<span class="nx">fmt</span><span class="p">.</span><span class="nx">Println</span><span class="p">(</span><span class="nx">msg</span><span class="p">)</span>
|
|
<span class="p">}</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
</table>
|
|
|
|
<table>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>When we run the program the <code>"ping"</code> message is
|
|
successfully passed from one goroutine to another via
|
|
our channel.</p>
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre><span class="gp">$</span> go run channels.go
|
|
<span class="go">ping</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>By default sends and receives block until both the
|
|
sender and receiver are ready. This property allowed
|
|
us to wait at the end of our program for the <code>"ping"</code>
|
|
message without having to use any other synchronization.</p>
|
|
|
|
</td>
|
|
<td class="code empty">
|
|
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
</table>
|
|
|
|
|
|
<p class="next">
|
|
Next example: <a href="channel-buffering">Channel Buffering</a>.
|
|
</p>
|
|
|
|
<p class="footer">
|
|
by <a href="https://markmcgranaghan.com">Mark McGranaghan</a> | <a href="https://github.com/mmcgrana/gobyexample/blob/master/examples/channels">source</a> | <a href="https://github.com/mmcgrana/gobyexample#license">license</a>
|
|
</p>
|
|
</div>
|
|
<script>
|
|
var codeLines = [];
|
|
codeLines.push('');codeLines.push('package main\u000A');codeLines.push('import \"fmt\"\u000A');codeLines.push('func main() {\u000A');codeLines.push(' messages := make(chan string)\u000A');codeLines.push(' go func() { messages \x3C- \"ping\" }()\u000A');codeLines.push(' msg := \x3C-messages\u000A fmt.Println(msg)\u000A}\u000A');codeLines.push('');codeLines.push('');
|
|
</script>
|
|
<script src="site.js" async></script>
|
|
</body>
|
|
</html>
|