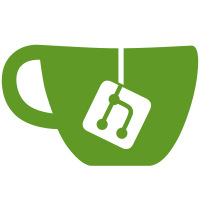
This bugfix implements correct way to calculate source sha1 hash, before it will changed, by propagation of unchanged sources. This commit will also include regenerated static files and *.hash files.
178 lines
6.7 KiB
Plaintext
Generated
178 lines
6.7 KiB
Plaintext
Generated
<!DOCTYPE html>
|
|
<html>
|
|
<head>
|
|
<meta charset="utf-8">
|
|
<title>Go by Example: Timers</title>
|
|
<link rel=stylesheet href="site.css">
|
|
</head>
|
|
<script>
|
|
onkeydown = (e) => {
|
|
|
|
if (e.key == "ArrowLeft") {
|
|
window.location.href = 'range-over-channels';
|
|
}
|
|
|
|
|
|
if (e.key == "ArrowRight") {
|
|
window.location.href = 'tickers';
|
|
}
|
|
|
|
}
|
|
</script>
|
|
<body>
|
|
<div class="example" id="timers">
|
|
<h2><a href="./">Go by Example</a>: Timers</h2>
|
|
|
|
<table>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>We often want to execute Go code at some point in the
|
|
future, or repeatedly at some interval. Go’s built-in
|
|
<em>timer</em> and <em>ticker</em> features make both of these tasks
|
|
easy. We’ll look first at timers and then
|
|
at <a href="tickers">tickers</a>.</p>
|
|
|
|
</td>
|
|
<td class="code empty leading">
|
|
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
<a href="http://play.golang.org/p/_cLT2ewHYO8"><img title="Run code" src="play.png" class="run" /></a><img title="Copy code" src="clipboard.png" class="copy" />
|
|
<div class="highlight"><pre><span class="kn">package</span> <span class="nx">main</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre><span class="kn">import</span> <span class="p">(</span>
|
|
<span class="s">"fmt"</span>
|
|
<span class="s">"time"</span>
|
|
<span class="p">)</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre><span class="kd">func</span> <span class="nx">main</span><span class="p">()</span> <span class="p">{</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>Timers represent a single event in the future. You
|
|
tell the timer how long you want to wait, and it
|
|
provides a channel that will be notified at that
|
|
time. This timer will wait 2 seconds.</p>
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre> <span class="nx">timer1</span> <span class="o">:=</span> <span class="nx">time</span><span class="p">.</span><span class="nx">NewTimer</span><span class="p">(</span><span class="mi">2</span> <span class="o">*</span> <span class="nx">time</span><span class="p">.</span><span class="nx">Second</span><span class="p">)</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>The <code><-timer1.C</code> blocks on the timer’s channel <code>C</code>
|
|
until it sends a value indicating that the timer
|
|
expired.</p>
|
|
|
|
</td>
|
|
<td class="code leading">
|
|
|
|
<div class="highlight"><pre> <span class="o"><-</span><span class="nx">timer1</span><span class="p">.</span><span class="nx">C</span>
|
|
<span class="nx">fmt</span><span class="p">.</span><span class="nx">Println</span><span class="p">(</span><span class="s">"Timer 1 expired"</span><span class="p">)</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>If you just wanted to wait, you could have used
|
|
<code>time.Sleep</code>. One reason a timer may be useful is
|
|
that you can cancel the timer before it expires.
|
|
Here’s an example of that.</p>
|
|
|
|
</td>
|
|
<td class="code">
|
|
|
|
<div class="highlight"><pre> <span class="nx">timer2</span> <span class="o">:=</span> <span class="nx">time</span><span class="p">.</span><span class="nx">NewTimer</span><span class="p">(</span><span class="nx">time</span><span class="p">.</span><span class="nx">Second</span><span class="p">)</span>
|
|
<span class="k">go</span> <span class="kd">func</span><span class="p">()</span> <span class="p">{</span>
|
|
<span class="o"><-</span><span class="nx">timer2</span><span class="p">.</span><span class="nx">C</span>
|
|
<span class="nx">fmt</span><span class="p">.</span><span class="nx">Println</span><span class="p">(</span><span class="s">"Timer 2 expired"</span><span class="p">)</span>
|
|
<span class="p">}()</span>
|
|
<span class="nx">stop2</span> <span class="o">:=</span> <span class="nx">timer2</span><span class="p">.</span><span class="nx">Stop</span><span class="p">()</span>
|
|
<span class="k">if</span> <span class="nx">stop2</span> <span class="p">{</span>
|
|
<span class="nx">fmt</span><span class="p">.</span><span class="nx">Println</span><span class="p">(</span><span class="s">"Timer 2 stopped"</span><span class="p">)</span>
|
|
<span class="p">}</span>
|
|
<span class="p">}</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
</table>
|
|
|
|
<table>
|
|
|
|
<tr>
|
|
<td class="docs">
|
|
<p>The first timer will expire ~2s after we start the
|
|
program, but the second should be stopped before it has
|
|
a chance to expire.</p>
|
|
|
|
</td>
|
|
<td class="code">
|
|
|
|
<div class="highlight"><pre><span class="gp">$</span> go run timers.go
|
|
<span class="go">Timer 1 expired</span>
|
|
<span class="go">Timer 2 stopped</span>
|
|
</pre></div>
|
|
|
|
</td>
|
|
</tr>
|
|
|
|
</table>
|
|
|
|
|
|
<p class="next">
|
|
Next example: <a href="tickers">Tickers</a>.
|
|
</p>
|
|
|
|
<p class="footer">
|
|
by <a href="https://markmcgranaghan.com">Mark McGranaghan</a> | <a href="https://github.com/mmcgrana/gobyexample/blob/master/examples/timers">source</a> | <a href="https://github.com/mmcgrana/gobyexample#license">license</a>
|
|
</p>
|
|
</div>
|
|
<script>
|
|
var codeLines = [];
|
|
codeLines.push('');codeLines.push('package main\u000A');codeLines.push('import (\u000A \"fmt\"\u000A \"time\"\u000A)\u000A');codeLines.push('func main() {\u000A');codeLines.push(' timer1 := time.NewTimer(2 * time.Second)\u000A');codeLines.push(' \x3C-timer1.C\u000A fmt.Println(\"Timer 1 expired\")\u000A');codeLines.push(' timer2 := time.NewTimer(time.Second)\u000A go func() {\u000A \x3C-timer2.C\u000A fmt.Println(\"Timer 2 expired\")\u000A }()\u000A stop2 := timer2.Stop()\u000A if stop2 {\u000A fmt.Println(\"Timer 2 stopped\")\u000A }\u000A}\u000A');codeLines.push('');
|
|
</script>
|
|
<script src="site.js" async></script>
|
|
</body>
|
|
</html>
|