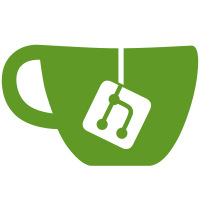
* [NOD-1500] Added Domain type and Constructor * [NOD-1500] Replaced dag+txpool with domain in flowContext * [NOD-1500] Replaced dag+txpool with domain in flowContext * [NOD-1500] Converters: domain objects from/to appmessage * [NOD-1500] Convert hashes to DomainHashes in appmessages * [NOD-1500] Remove references to daghash in dagconfig * [NOD-1500] Fixed all appmessage usages of hashes * [NOD-1500] Update all RPC to use domain * [NOD-1500] Big chunk of protocol flows re-wired to domain * [NOD-1500] Finished re-wiring all protocol flows to new Domain * [NOD-1500] Fix some mempool and kaspaminer compilation errors * [NOD-1500] Deleted util/{block,tx,daghash} and dbaccess * [NOD-1500] util.CoinbaseTransactionIndex -> transactionhelper.CoinbaseTransactionIndex * [NOD-1500] Fix txsigner * [NOD-1500] Removed all references to util/subnetworkid * [NOD-1500] Update RpcGetBlock related messages * [NOD-1500] Many more compilation fixes * [NOD-1500] Return full list of missing blocks for orphan resolution * [NOD-1500] Fixed handshake * [NOD-1500] Fixed flowcontext compilation * [NOD-1500] Update users of StartIBDIfRequired to handle error * [NOD-1500] Removed some more fields from RPC * [NOD-1500] Fix the getBlockTemplate flow * [NOD-1500] Fix HandleGetCurrentNetwork * [NOD-1500] Remove redundant code * [NOD-1500] Remove obsolete notifications * [NOD-1500] Split MiningManager and Consensus to separate fields in Domain * [NOD-1500] Update two wrong references to location of txscript * [NOD-1500] Added comments * [NOD-1500] Fix some tests * [NOD-1500] Removed serialization logic from appmessage * [NOD-1500] Rename database/serialization/messages.proto to dbobjects.proto * [NOD-1500] Delete integration tests * [NOD-1500] Remove txsort * [NOD-1500] Fix tiny bug * [NOD-1500] Remove rogue dependancy on bchd * [NOD-1500] Some stylistic fixes
wire
Package wire implements the kaspa wire protocol.
Kaspa Message Overview
The kaspa protocol consists of exchanging messages between peers. Each message is preceded by a header which identifies information about it such as which kaspa network it is a part of, its type, how big it is, and a checksum to verify validity. All encoding and decoding of message headers is handled by this package.
To accomplish this, there is a generic interface for kaspa messages named
Message
which allows messages of any type to be read, written, or passed
around through channels, functions, etc. In addition, concrete implementations
of most all kaspa messages are provided. All of the details of marshalling and
unmarshalling to and from the wire using kaspa encoding are handled so the
caller doesn't have to concern themselves with the specifics.
Reading Messages Example
In order to unmarshal kaspa messages from the wire, use the ReadMessage
function. It accepts any io.Reader
, but typically this will be a net.Conn
to a remote node running a kaspa peer. Example syntax is:
// Use the most recent protocol version supported by the package and the
// main kaspa network.
pver := wire.ProtocolVersion
kaspanet := wire.Mainnet
// Reads and validates the next kaspa message from conn using the
// protocol version pver and the kaspa network kaspanet. The returns
// are a appmessage.Message, a []byte which contains the unmarshalled
// raw payload, and a possible error.
msg, rawPayload, err := wire.ReadMessage(conn, pver, kaspanet)
if err != nil {
// Log and handle the error
}
See the package documentation for details on determining the message type.
Writing Messages Example
In order to marshal kaspa messages to the wire, use the WriteMessage
function. It accepts any io.Writer
, but typically this will be a net.Conn
to a remote node running a kaspa peer. Example syntax to request addresses
from a remote peer is:
// Use the most recent protocol version supported by the package and the
// main bitcoin network.
pver := wire.ProtocolVersion
kaspanet := wire.Mainnet
// Create a new getaddr kaspa message.
msg := wire.NewMsgGetAddr()
// Writes a kaspa message msg to conn using the protocol version
// pver, and the kaspa network kaspanet. The return is a possible
// error.
err := wire.WriteMessage(conn, msg, pver, kaspanet)
if err != nil {
// Log and handle the error
}