mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
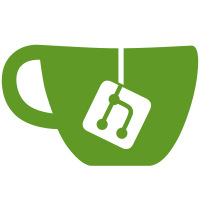
* [NOD-1500] Added Domain type and Constructor * [NOD-1500] Replaced dag+txpool with domain in flowContext * [NOD-1500] Replaced dag+txpool with domain in flowContext * [NOD-1500] Converters: domain objects from/to appmessage * [NOD-1500] Convert hashes to DomainHashes in appmessages * [NOD-1500] Remove references to daghash in dagconfig * [NOD-1500] Fixed all appmessage usages of hashes * [NOD-1500] Update all RPC to use domain * [NOD-1500] Big chunk of protocol flows re-wired to domain * [NOD-1500] Finished re-wiring all protocol flows to new Domain * [NOD-1500] Fix some mempool and kaspaminer compilation errors * [NOD-1500] Deleted util/{block,tx,daghash} and dbaccess * [NOD-1500] util.CoinbaseTransactionIndex -> transactionhelper.CoinbaseTransactionIndex * [NOD-1500] Fix txsigner * [NOD-1500] Removed all references to util/subnetworkid * [NOD-1500] Update RpcGetBlock related messages * [NOD-1500] Many more compilation fixes * [NOD-1500] Return full list of missing blocks for orphan resolution * [NOD-1500] Fixed handshake * [NOD-1500] Fixed flowcontext compilation * [NOD-1500] Update users of StartIBDIfRequired to handle error * [NOD-1500] Removed some more fields from RPC * [NOD-1500] Fix the getBlockTemplate flow * [NOD-1500] Fix HandleGetCurrentNetwork * [NOD-1500] Remove redundant code * [NOD-1500] Remove obsolete notifications * [NOD-1500] Split MiningManager and Consensus to separate fields in Domain * [NOD-1500] Update two wrong references to location of txscript * [NOD-1500] Added comments * [NOD-1500] Fix some tests * [NOD-1500] Removed serialization logic from appmessage * [NOD-1500] Rename database/serialization/messages.proto to dbobjects.proto * [NOD-1500] Delete integration tests * [NOD-1500] Remove txsort * [NOD-1500] Fix tiny bug * [NOD-1500] Remove rogue dependancy on bchd * [NOD-1500] Some stylistic fixes
89 lines
2.5 KiB
Go
89 lines
2.5 KiB
Go
// Copyright (c) 2013-2016 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package appmessage
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/davecgh/go-spew/spew"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/util/mstime"
|
|
"github.com/kaspanet/kaspad/util/random"
|
|
)
|
|
|
|
// TestBlockHeader tests the BlockHeader API.
|
|
func TestBlockHeader(t *testing.T) {
|
|
nonce, err := random.Uint64()
|
|
if err != nil {
|
|
t.Errorf("random.Uint64: Error generating nonce: %v", err)
|
|
}
|
|
|
|
hashes := []*externalapi.DomainHash{mainnetGenesisHash, simnetGenesisHash}
|
|
|
|
merkleHash := mainnetGenesisMerkleRoot
|
|
acceptedIDMerkleRoot := exampleAcceptedIDMerkleRoot
|
|
bits := uint32(0x1d00ffff)
|
|
bh := NewBlockHeader(1, hashes, merkleHash, acceptedIDMerkleRoot, exampleUTXOCommitment, bits, nonce)
|
|
|
|
// Ensure we get the same data back out.
|
|
if !reflect.DeepEqual(bh.ParentHashes, hashes) {
|
|
t.Errorf("NewBlockHeader: wrong prev hashes - got %v, want %v",
|
|
spew.Sprint(bh.ParentHashes), spew.Sprint(hashes))
|
|
}
|
|
if bh.HashMerkleRoot != merkleHash {
|
|
t.Errorf("NewBlockHeader: wrong merkle root - got %v, want %v",
|
|
spew.Sprint(bh.HashMerkleRoot), spew.Sprint(merkleHash))
|
|
}
|
|
if bh.Bits != bits {
|
|
t.Errorf("NewBlockHeader: wrong bits - got %v, want %v",
|
|
bh.Bits, bits)
|
|
}
|
|
if bh.Nonce != nonce {
|
|
t.Errorf("NewBlockHeader: wrong nonce - got %v, want %v",
|
|
bh.Nonce, nonce)
|
|
}
|
|
}
|
|
|
|
func TestIsGenesis(t *testing.T) {
|
|
nonce := uint64(123123) // 0x1e0f3
|
|
bits := uint32(0x1d00ffff)
|
|
timestamp := mstime.UnixMilliseconds(0x495fab29000)
|
|
|
|
baseBlockHdr := &BlockHeader{
|
|
Version: 1,
|
|
ParentHashes: []*externalapi.DomainHash{mainnetGenesisHash, simnetGenesisHash},
|
|
HashMerkleRoot: mainnetGenesisMerkleRoot,
|
|
Timestamp: timestamp,
|
|
Bits: bits,
|
|
Nonce: nonce,
|
|
}
|
|
genesisBlockHdr := &BlockHeader{
|
|
Version: 1,
|
|
ParentHashes: []*externalapi.DomainHash{},
|
|
HashMerkleRoot: mainnetGenesisMerkleRoot,
|
|
Timestamp: timestamp,
|
|
Bits: bits,
|
|
Nonce: nonce,
|
|
}
|
|
|
|
tests := []struct {
|
|
in *BlockHeader // Block header to encode
|
|
isGenesis bool // Expected result for call of .IsGenesis
|
|
}{
|
|
{genesisBlockHdr, true},
|
|
{baseBlockHdr, false},
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
isGenesis := test.in.IsGenesis()
|
|
if isGenesis != test.isGenesis {
|
|
t.Errorf("BlockHeader.IsGenesis: #%d got: %t, want: %t",
|
|
i, isGenesis, test.isGenesis)
|
|
}
|
|
}
|
|
}
|